Create react components with ai
Explore how to generate Login component using v0 AI with React and Tailwind ready.
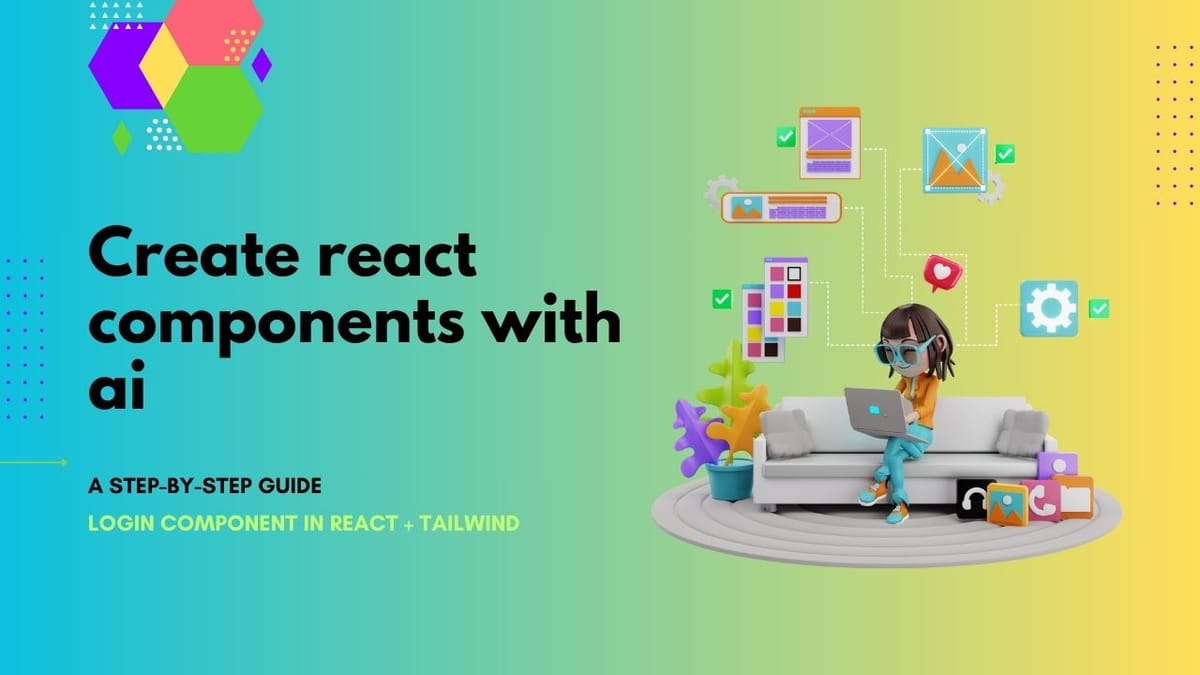
AI is taking a leap day by day in all the industry but there is major impact today in IT industry especially in software development. Let's understand how to build react component using AI that generates react and tailwind ready component.
Output from AI
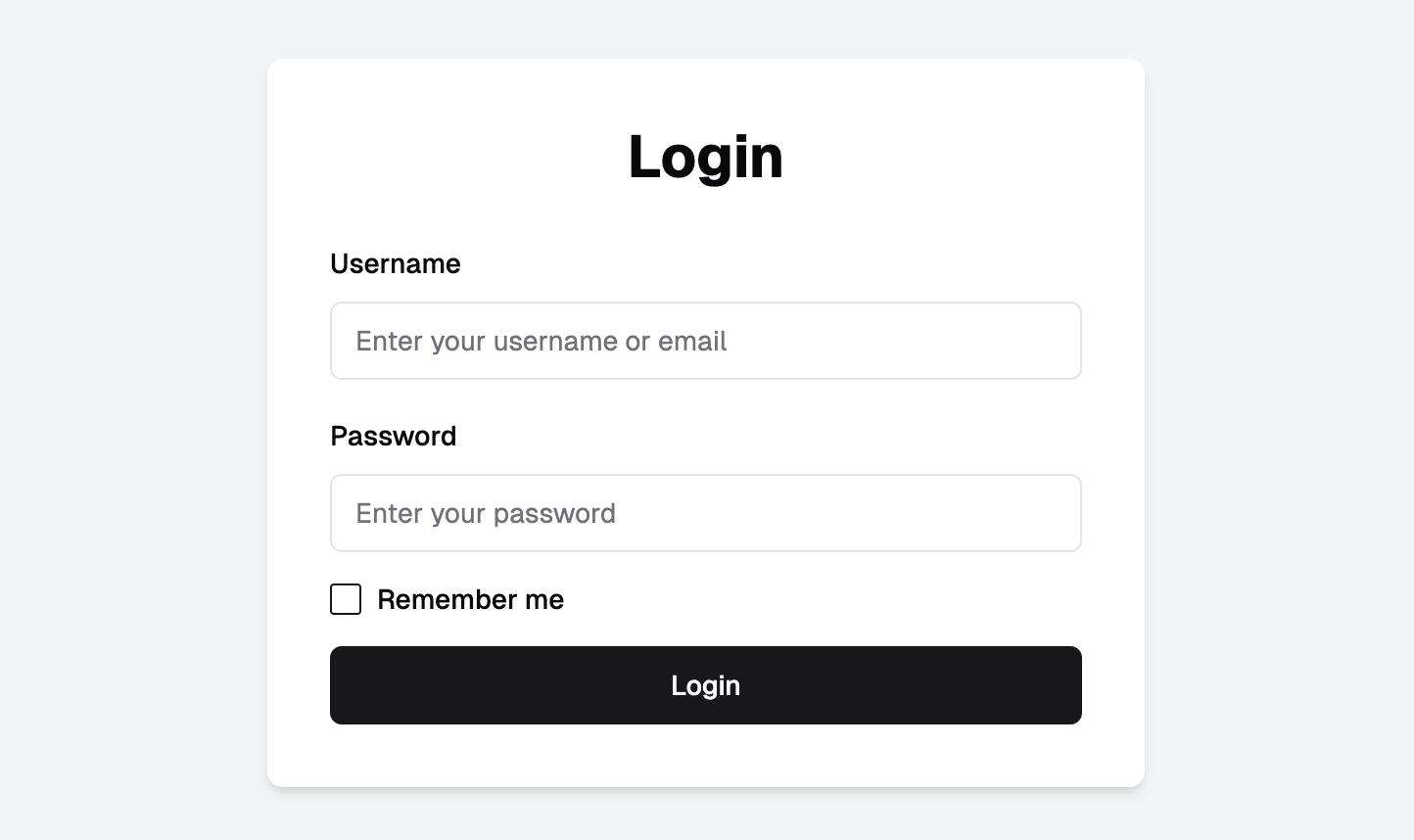
Let's delve deep how I made this with AI in 30 seconds
Let's understand What is v0?
v0 is generative ai for user interface model built by Vercel, Let's say prompt to react + tailwind + shadcn component ready!
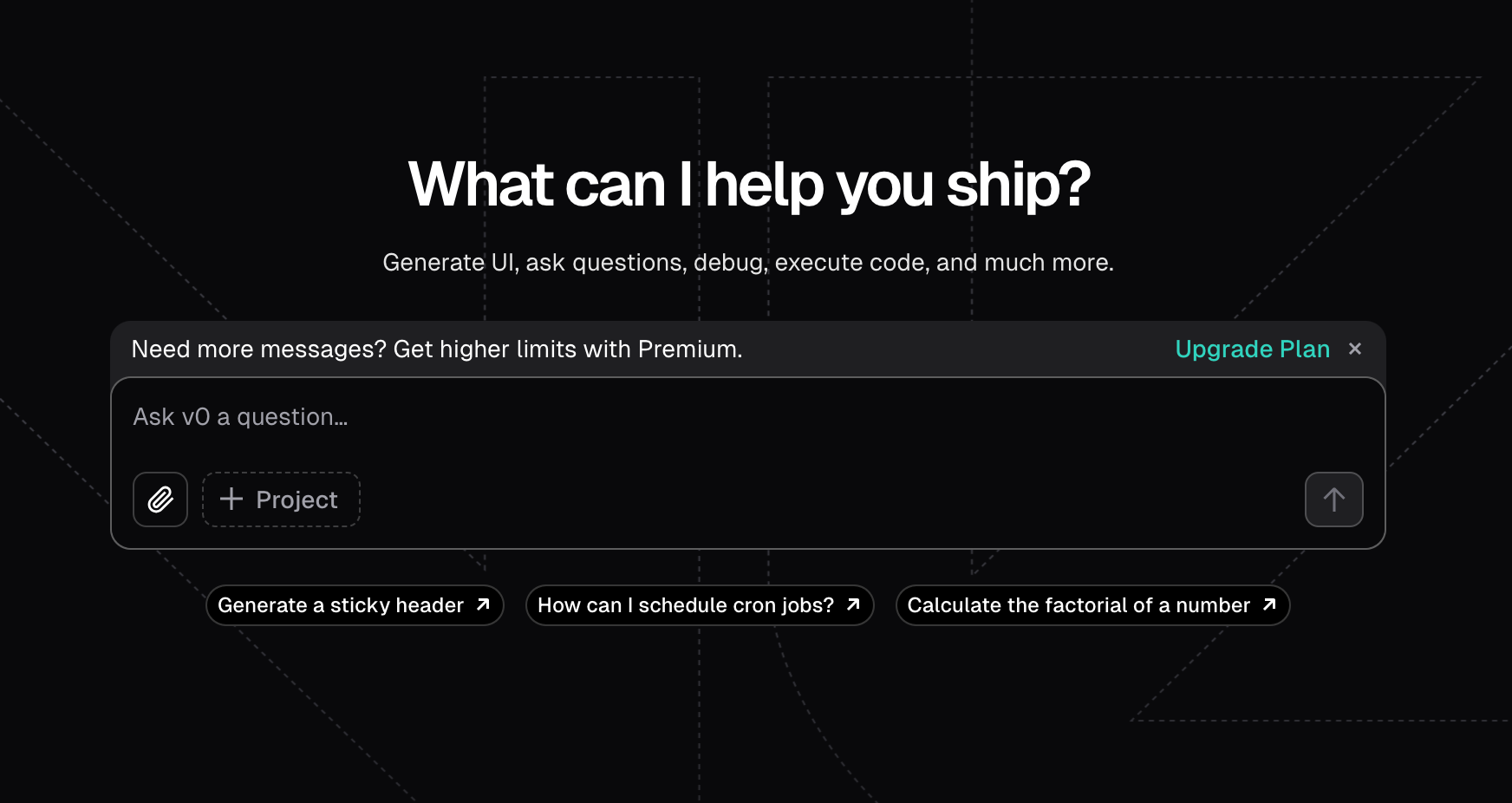
Go to https://v0.dev
Prompt in action
Generate a login component that has username, password, remember me, submit button component, Fields: Username, Password, remember me Validations username: required, alphanumeric or email, password: alphanumeric and last character should be number Schema validation: Zod Create onSubmit function with sample post fetch request with async and await in try catch block and handle error and loading state. UI: use shadcn ui inputs and buttons Align this component in center of the page
Prompt breakdown
- Generate a login component that has username, password, remember me, submit button component.
- Fields: Username, Password, remember me
- Validations username: required, alphanumeric or email, password: alphanumeric and last character should number
- Schema validation: Zod
- Create onSubmit function with sample post fetch request with async and await in try catch block and handle error and loading state.
- UI: use shadcn ui inputs and buttons
- Preview: Align this component in center of the page
Response from v0
I'll create a login component with the specifications you've requested. This component will use Zod for schema validation, shadcn UI components, and include a sample POST fetch request with error and loading state handling.
This login component includes the following features:
- Username and password fields with the required validations using Zod.
- A "Remember me" checkbox.
- Form validation using react-hook-form with Zod resolver.
- A submit button that shows a loading state during form submission.
- An asynchronous onSubmit function with a sample POST fetch request, wrapped in a try-catch block to handle errors.
- Error and success notifications using the toast component from shadcn UI.
- Styled using shadcn UI components (Input, Button, Checkbox, Label) and centered on the page.
To use this component, you'll need to have the shadcn UI components installed and configured in your project. You'll also need to install the following dependencies:
- zod
- react-hook-form
- @hookform/resolvers
Make sure to adjust the import paths for the shadcn UI components if necessary, depending on your project structure.
This component provides a solid foundation for a login form with proper validation, error handling, and user feedback. You can further customize it to fit your specific needs or styling preferences.
Code Output
'use client'
import { useState } from 'react'
import { z } from 'zod'
import { useForm } from 'react-hook-form'
import { zodResolver } from '@hookform/resolvers/zod'
import { Input } from "@/components/ui/input"
import { Button } from "@/components/ui/button"
import { Checkbox } from "@/components/ui/checkbox"
import { Label } from "@/components/ui/label"
import { useToast } from "@/components/ui/use-toast"
// Define the Zod schema for form validation
const loginSchema = z.object({
username: z.string().min(1, 'Username is required').regex(/^[a-zA-Z0-9@.]+$/, 'Username must be alphanumeric or an email'),
password: z.string().min(1, 'Password is required').regex(/^[a-zA-Z0-9]+$/, 'Password must be alphanumeric'),
rememberMe: z.boolean().optional()
})
type LoginFormData = z.infer<typeof loginSchema>
export default function LoginForm() {
const [isLoading, setIsLoading] = useState(false)
const { toast } = useToast()
const { register, handleSubmit, formState: { errors } } = useForm<LoginFormData>({
resolver: zodResolver(loginSchema)
})
const onSubmit = async (data: LoginFormData) => {
setIsLoading(true)
try {
const response = await fetch('/api/login', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
},
body: JSON.stringify(data),
})
if (!response.ok) {
throw new Error('Login failed')
}
const result = await response.json()
toast({
title: "Success",
description: "You have successfully logged in.",
})
// Handle successful login (e.g., redirect, update state, etc.)
} catch (error) {
toast({
title: "Error",
description: "Login failed. Please try again.",
variant: "destructive",
})
} finally {
setIsLoading(false)
}
}
return (
<div className="flex items-center justify-center min-h-screen bg-gray-100">
<div className="w-full max-w-md p-8 space-y-6 bg-white rounded-lg shadow-md">
<h2 className="text-3xl font-bold text-center">Login</h2>
<form onSubmit={handleSubmit(onSubmit)} className="space-y-4">
<div className="space-y-2">
<Label htmlFor="username">Username</Label>
<Input
id="username"
type="text"
placeholder="Enter your username or email"
{...register('username')}
/>
{errors.username && (
<p className="text-sm text-red-500">{errors.username.message}</p>
)}
</div>
<div className="space-y-2">
<Label htmlFor="password">Password</Label>
<Input
id="password"
type="password"
placeholder="Enter your password"
{...register('password')}
/>
{errors.password && (
<p className="text-sm text-red-500">{errors.password.message}</p>
)}
</div>
<div className="flex items-center space-x-2">
<Checkbox id="rememberMe" {...register('rememberMe')} />
<Label htmlFor="rememberMe">Remember me</Label>
</div>
<Button type="submit" className="w-full" disabled={isLoading}>
{isLoading ? 'Logging in...' : 'Login'}
</Button>
</form>
</div>
</div>
)
}
Preview
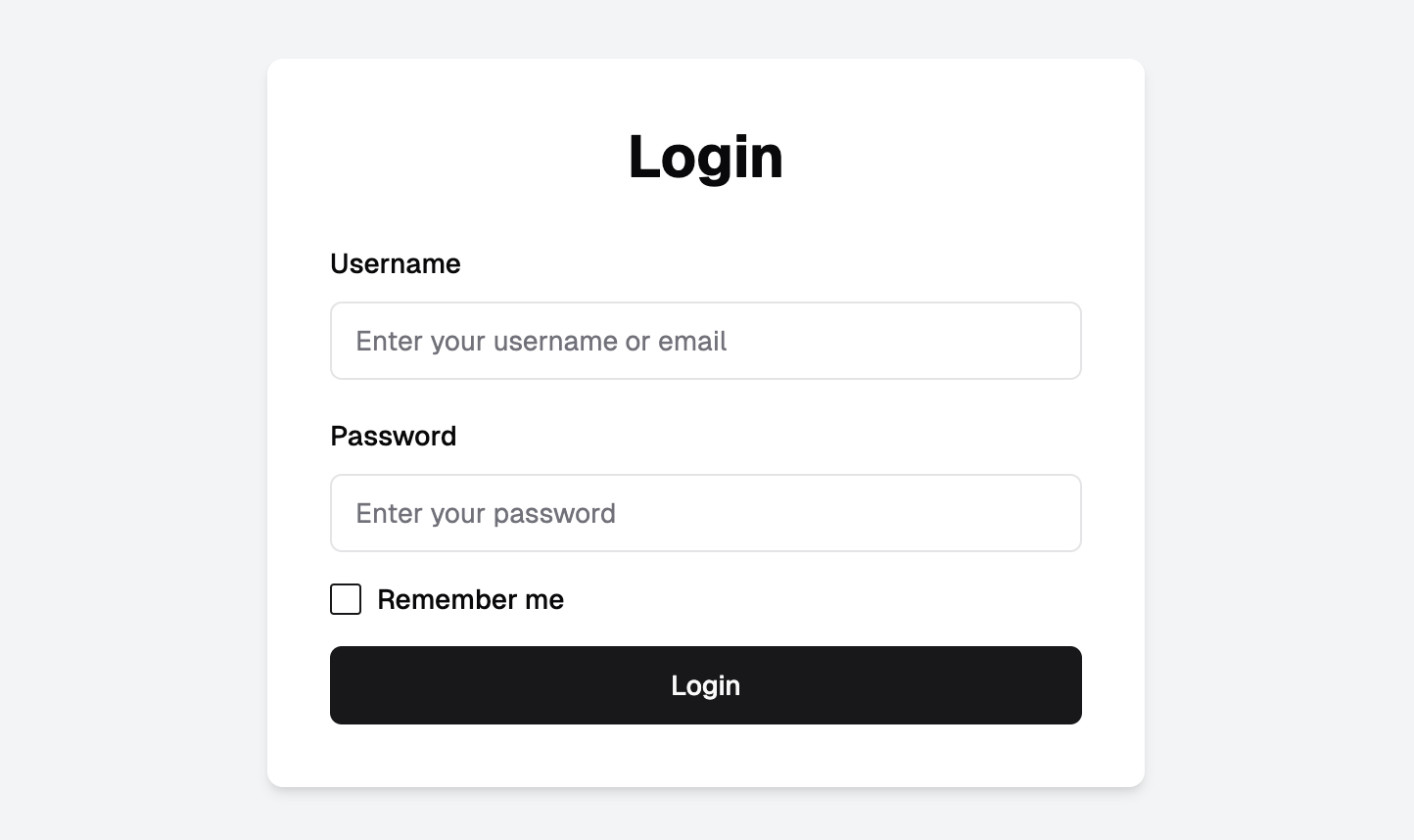
It took only 30 seconds, to generate this react ready component from v0, in future this will be more optimised and concise.
Alright, Subscribe for more content like this.